sebastiandaschner blog
JPA joined subclass strategy example
wednesday, april 08, 2015JPA supports several ways to map class hierarchies to the database. For a hierarchy with two or more classes which need their own tables, the joined subclass strategy can be used.
Imagine following model:
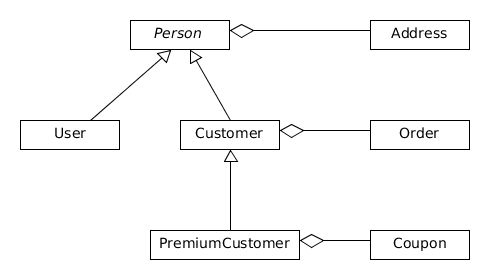
These classes are mapped to own database tables as every table has to be mapped from another entity (like Order
or Address
for example) — so MappedSuperclass
wouldn’t work in this example.
The joined subclass strategy implies that the fields of each class in the hierarchy are placed in their own tables and that the tables are joined to construct the result.
The classes would look like follows:
@Entity
@Table(name = "persons")
@Inheritance(strategy = InheritanceType.JOINED)
public abstract class Person {
@Id
@GeneratedValue(strategy = GenerationType.SEQUENCE, generator = "person_seq")
@SequenceGenerator(name = "person_seq", sequenceName = "person_seq")
private long id;
@Basic(optional = false)
private String name;
@OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.ALL, orphanRemoval = true)
@JoinColumn(name = "person", nullable = false)
private Set<Address> addresses;
// ...
}
@Entity
@Table(name = "customers")
@PrimaryKeyJoinColumn(name = "person")
public class Customer extends Person {
@OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.ALL, orphanRemoval = true)
@JoinColumn(name = "customer_order", nullable = true)
private Set<Order> orders = new HashSet<>();
// ...
}
@Entity
@Table(name = "premium_customers")
@PrimaryKeyJoinColumn(name = "customer")
public class PremiumCustomer extends Customer {
@OneToMany(fetch = FetchType.LAZY, cascade = CascadeType.ALL, orphanRemoval = true, mappedBy = "premiumCustomer")
private Set<Coupon> coupons = new HashSet<>();
// ...
}
@Entity
@Table(name = "application_users")
@PrimaryKeyJoinColumn(name = "person")
public class User extends Person {
// ...
}
The PrimaryKeyJoinColumn
is included as foreign key which references the primary key of the direct superclass.
Found the post useful? Subscribe to my newsletter for more free content, tips and tricks on IT & Java: