sebastiandaschner blog
Neo4J OGM with Quarkus (Video)
monday, may 18, 2020In the following video I show an example Quarkus application that uses a Neo4J database and Neo4J OGM.
Have a look at the example project on GitHub.
I’ve created an example domain for coffee beans with certain flavor profiles that we can query and match. The red nodes are coffee beans that are from a certain country and taste like certain flavors. Bean origin countries are also “known for” certain flavors.
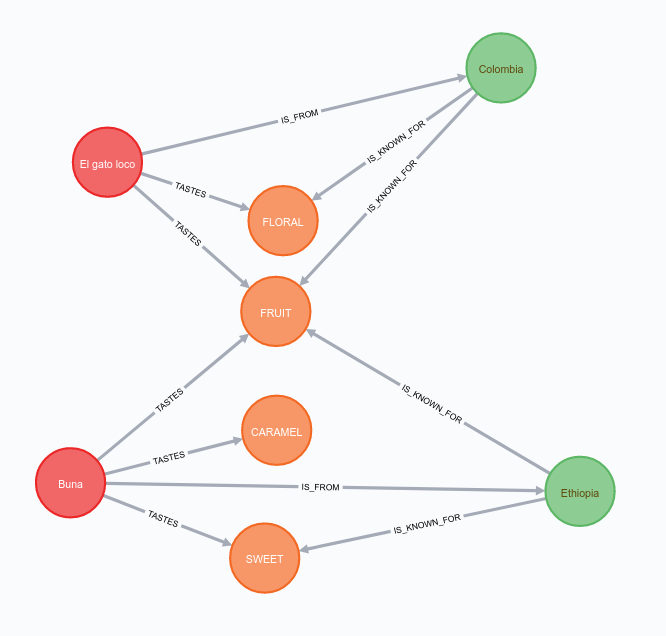
In the current version 1.4.2.Final
, Quarkus comes with basic Neo4J support, but as of writing this not with included support for OGM mapping.
However, we can add the support with one simple producer that exposes a Neo4J OGM SessionFactory
:
import org.neo4j.ogm.config.Configuration;
import org.neo4j.ogm.session.SessionFactory;
...
@ApplicationScoped
public class SessionFactoryProducer {
public static final String PACKAGE = "com.sebastian_daschner.coffee.entity";
@ConfigProperty(name = "quarkus.neo4j.uri")
String databaseUri;
@ConfigProperty(name = "quarkus.neo4j.authentication.username")
String username;
@ConfigProperty(name = "quarkus.neo4j.authentication.password")
String password;
@Produces
SessionFactory produceSessionFactory() {
Configuration neoConfig = new Configuration.Builder()
.uri(databaseUri)
.credentials(username, password)
.useNativeTypes()
.build();
return new SessionFactory(neoConfig, PACKAGE);
}
void disposeSessionFactory(@Disposes SessionFactory sessionFactory) {
sessionFactory.close();
}
}
Now we can inject the SessionFactory
in our beans and use it to query our graph database:
import org.neo4j.ogm.session.*;
...
@ApplicationScoped
public class CoffeeBeans {
@Inject
SessionFactory sessionFactory;
public List<CoffeeBean> getCoffeeBeans() {
Session session = sessionFactory.openSession();
return new ArrayList<>(session.loadAll(CoffeeBean.class,
new SortOrder("name"), 1));
}
public List<CoffeeBean> getCoffeeBeansSpecificFlavor(String flavor) {
Session session = sessionFactory.openSession();
Iterable<CoffeeBean> result = session.query(CoffeeBean.class,
"MATCH (b:CoffeeBean)-[:TASTES]->(:Flavor {description: $flavor})\n" +
"MATCH (b)-[isFrom:IS_FROM]->(country)\n" +
"MATCH (b)-[tastes:TASTES]->(flavor)\n" +
"RETURN b, collect(isFrom), collect(country)," +
" collect(tastes), collect(flavor)\n" +
"ORDER by b.name;",
Map.of("flavor", flavor));
return resultList(result);
}
...
}
My example graph looks as follows, with the CoffeeBean
objects being mapped via Neo4J OGM:
import org.neo4j.ogm.annotation.*;
...
@NodeEntity
public class CoffeeBean {
@Id
public String name;
@Relationship("IS_FROM")
public Set<Origin> origins = new HashSet<>();
@Property
public Roast roast;
@Relationship("TASTES")
public Set<FlavorProfile> flavorProfiles = new HashSet<>();
...
}
Watch the video and have a look at the project on GitHub to get the full picture.
Have fun exploring your graph! As some further exercise, you can write queries that match coffee beans that have a certain flavor as their “main” flavor (the one with the highest percentage), or beans with similar flavor profiles, etc.
Found the post useful? Subscribe to my newsletter for more free content, tips and tricks on IT & Java: