sebastiandaschner blog
Controlling Elgato Keylights From the Command Line
monday, february 06, 2023If you own Elgato Keylights for your video setup, you can use on of their apps to setup and control, or you can use the command line. For setting up new lights or tweaking the brightness or warmth, it might make sense to use an official app, while for quickly turning all of them on or off, a quick command line script or alias might me more effective.
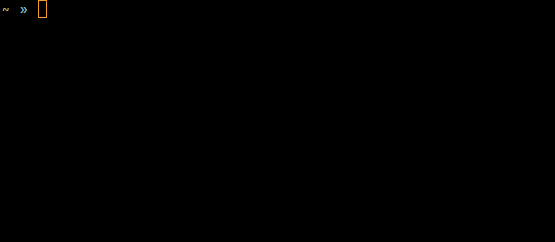
The Keylights actually expose an HTTP API that we can use via curl
or the HTTP client of your choice:
$> curl 192.168.1.111:9123/elgato/lights | jq . { "numberOfLights": 1, "lights": [ { "on": 0, "brightness": 36, "temperature": 255 } ] }
You can control the lights by updating the desired state, which is what the elgato
script does, that you can find in my Dotfiles.
The only missing link is how to find out the lights' addresses. The official apps discover them at startup in your local network, which always takes a short moment. Thus, if you have a stable light and network setup, it’s more efficient to cache the addresses locally.
You can discover the addresses yourself via mDNS and the _elg._tcp
type.
Under Linux this works using the avahi
tool:
$> sudo avahi-daemon # in a new terminal $> avahi-browse -t _elg._tcp --resolve + enp10s0 IPv6 Elgato Key Light XXXX _elg._tcp local = enp10s0 IPv6 Elgato Key Light XXXX _elg._tcp local hostname = [elgato-key-light-XXXX.local] address = [192.168.1.111] port = [9123] txt = ["pv=1.0" "md=Elgato Key Light XXX" "id=XX:XX:XX:XX:XX:XX" "dt=53" "mf=Elgato"] [...]
You can take take note of the address
and port
.
Or, if you prefer a Java solution to query the addresses, you can use the following code:
import javax.jmdns.JmDNS;
import javax.jmdns.ServiceInfo;
import java.io.IOException;
import java.net.InetAddress;
public class Resolver {
public static void main(String[] args) throws IOException {
JmDNS jmdns = JmDNS.create(InetAddress.getLocalHost());
ServiceInfo[] elgatoServices = jmdns.list("_elg._tcp.local.");
for (ServiceInfo elgatoService : elgatoServices) {
System.out.println("elgato = "
+ elgatoService.getInet4Addresses()[0].getHostAddress()
+ ':' + elgatoService.getPort());
}
}
}
The jmDNS dependency is added with the following Maven coordinates:
<dependency>
<groupId>org.jmdns</groupId>
<artifactId>jmdns</artifactId>
<version>3.5.8</version>
</dependency>
Running the Resolver
class gives you basically the same result:
elgato = 192.168.1.111:9123 elgato = 192.168.1.112:9123
Now that you have the addresses, you can put them into an environment variable or .env
file and control the lights on the command line.
To see how to put that together, have a look at the elgato
script in my Dotfiles as an example.
Found the post useful? Subscribe to my newsletter for more free content, tips and tricks on IT & Java: